本文共 3513 字,大约阅读时间需要 11 分钟。
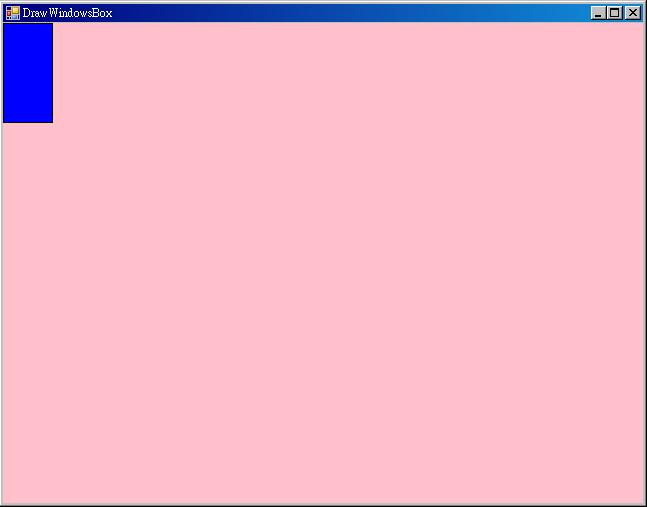
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using Microsoft.DirectX;
using Microsoft.DirectX.DirectDraw;

namespace DrectDrawLab
{ 
public partial class DrawWindowsBox : Form
{
// window width & window height
int _width = 640;
int _height = 480;
// DirectDraw Device
Device _device = null;
// Clipper
Clipper _clipper = null;
// Primary Surface
Surface _primarySurface = null;
// Secondary Surface
Surface _secondarySurface = null;

public static void Main()
{
DrawWindowsBox frm = new DrawWindowsBox();
Application.Exit();
}

public DrawWindowsBox()
{
InitializeComponent();
// Create DirectDraw Device
this.createDevice();
// Create Clipper
this.createClipper();
// Create Primary Surface
this.createPrimarySurface();
// Create Secondary Surface
this.createSecondarySurface();
// Show the window
this.Show();
// Start message loop
this.startLoop();
}
// Step 1. Device Creation
void createDevice()
{
// Create new DirectDraw device
_device = new Device();
// set windowed mode, owner is this form
_device.SetCooperativeLevel(this, CooperativeLevelFlags.Normal);
}
// Step 2.Clipper Creation
void createClipper()
{
// Create new clipper
_clipper = new Clipper(_device);
// Set window size
this.ClientSize = new Size(_width, _height);
// Set window associated to clipper
_clipper.Window = this;
}
// Step 3.Primary Surface Creation
void createPrimarySurface()
{
// Create new SurfaceDescription to create Surface
SurfaceDescription desc = new SurfaceDescription();
// Use System Memory
desc.SurfaceCaps.SystemMemory = true;
// To create Primary Surface
desc.SurfaceCaps.PrimarySurface = true;
// Create new Primary Surface
_primarySurface = new Surface(desc, _device);
// Set clipper associated to surface
_primarySurface.Clipper = _clipper;
}
// Step 4.Secondary Surface Creation
void createSecondarySurface()
{
// Create new SurfaceDescription to create Surface
SurfaceDescription desc = new SurfaceDescription();
// Use System Memory
desc.SurfaceCaps.SystemMemory = true;
// To create Secondary Surface
desc.SurfaceCaps.OffScreenPlain = true;
// Set Secondary Surface width as Primary Surface width
desc.Width = _primarySurface.SurfaceDescription.Width;
// Set Secondary Surface height as Primary Surface height
desc.Height = _primarySurface.SurfaceDescription.Height;
// Crate Secondary Surface
_secondarySurface = new Surface(desc, _device);
// Create new Clipper to Secondary Surface
_secondarySurface.Clipper = new Clipper();
// Create Clipper List
_secondarySurface.Clipper.ClipList = new Rectangle[]
{ new Rectangle(0, 0, _width, _height) };
}
// Step 5.Draw line
void renderGraphics()
{
if (!this.Created)
return;
if (_primarySurface == null || _secondarySurface == null)
return;
// Fill back color
_secondarySurface.ColorFill(Color.Pink);
// Fill fore color
_secondarySurface.FillColor = Color.Blue;
// Draw Box by DirectDraw
_secondarySurface.DrawBox(0, 0, 50, 100);
// Draw Primary Surface by Secondary Surface
Rectangle srcRect = new Rectangle(0, 0, _width, _height);
_primarySurface.Draw(this.RectangleToScreen(this.ClientRectangle), _secondarySurface, srcRect, DrawFlags.DoNotWait);
}
// Message Loop
void startLoop()
{ 
while (this.Created)
{
this.renderGraphics();
Application.DoEvents();
}
}

private void DrawWindowsBox_Activated(object sender, EventArgs e)
{
this.renderGraphics();
}

private void DrawWindowsBox_Resize(object sender, EventArgs e)
{
this.renderGraphics();
}
}
} 转载地址:http://famyo.baihongyu.com/